Home »
Android
How to use bundle class for passing data of any type in Android?
Bundle Class in Android with Example: In this tutorial, we will learn how to use bundle class to pass data between activities and initializes the key for the values we pass.
By Manu Jemini Last updated : June 06, 2023
Bundle Class
A bundle is used to move data from one activity to another. This is very helpful when you need some data in an activity that will come from another activity.
Android Bundle Class Example
In the example below, we have implemented two activities, namely MainActivity and SecondActivity. First, let us understand what we are doing here.
We are using the First layout to show two EditTexts two have a nice looking interface for our user. We have an onClick listener which is used to invoke the class OnClickListener, which in turn takes the values from the EditTexts and save them in the strings. These string namely field1 and field2 will be the values of the EditTexts.
To switch the control from one activity to another activity we need Intent. So to initialize the Intent class object we use this line.
Intent I=new Intent(MainActivity.this, SecondActivity.class);
The First parameter to the constructor is the context of the current activity and the second parameter is the class of the activity to which we want to go.
Bundle b = new Bundle();
b.putString("dataone",data1);
b.putString("datatwo",data2);
Here this is making an object of Bundle class and also putting the data fields we have from the EditTexts.
The Only thing left is to put the bundle with Intent object and start the activity.
I.putExtras(b);
startActivity(I);
In the SecondActivity, we take our strings out from the Intent and use the values to change the content of TextViews of second layout file.
Bundle b= getIntent().getExtras();
Java file (1)
package com.example.hp.demo;
import android.content.Context;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity
{
EditText field1,field2;
Button B;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
field1=(EditText)findViewById(R.id.field1);
field2=(EditText)findViewById(R.id.field2);
B = (Button) findViewById(R.id.button);
B.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String data1=field1.getText().toString();
String data2=field2.getText().toString();
Intent I=new Intent(MainActivity.this, SecondActivity.class);
Bundle b = new Bundle();
b.putString("dataone",data1);
b.putString("datatwo",data2);
I.putExtras(b);
startActivity(I);
}});
}
}
Java file (2)
package com.example.hp.demo;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextView;
public class SecondActivity extends AppCompatActivity {
TextView textview;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_second);
textview=(TextView)findViewById(R.id.textView);
Bundle b= getIntent().getExtras();
String data1= b.getString("dataone");
String data2= b.getString("datatwo");
textview.setText("Field1 : "+data1+"\nField2 : "+data2);
}
}
Xml file (1)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:weightSum="1">
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/field1"
android:layout_alignParentStart="true"
android:layout_alignParentEnd="true"
android:hint="field1" />
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:inputType="phone"
android:ems="10"
android:id="@+id/field2"
android:layout_below="@+id/field1"
android:layout_alignParentStart="true"
android:layout_alignParentEnd="true"
android:hint="field2" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click"
android:id="@+id/button"
android:layout_below="@+id/field2"
android:layout_alignParentStart="true" />
</RelativeLayout>
Xml file (2)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.hp.demo.SecondActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="100dp"
android:id="@+id/textView"
android:layout_alignParentTop="true"
android:layout_alignParentStart="true"
android:layout_alignParentEnd="true" />
</RelativeLayout>
Android manifest
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.hp.demo">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SecondActivity"></activity>
</application>
</manifest>
Output
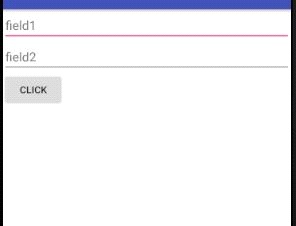