Home »
JQuery
JQuery HTML
In this tutorial, we will explore JQuery further by understanding how to use JQuery Selectors to change content or HTML of a page?
Submitted by Siddhant Verma, on November 16, 2019
If you're not familiar with JQuery selectors, have a quick read through here: JQuery selectors.
We'll learn how to query the DOM using selectors using JQuery selectors by building this simple and awesome mini Pokedex so let's get started. I'll be using the same boilerplate with a CDN to JQuery and MaterializeCSS. Let's create a template to get started,
index.html
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<!-- Compiled and minified CSS -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/css/materialize.min.css">
<!-- Compiled and minified JavaScript -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/1.0.0/js/materialize.min.js"></script>
<title>Pokedex</title>
<style>
body {
background: whitesmoke;
}
.card-image img {
height: 200px;
width: 200px;
}
</style>
</head>
<body>
<div class="container">
<h1 class="center">My Pokedex</h1>
<div class="row">
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://assets.pokemon.com/assets/cms2/img/pokedex/full/007.png">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://giantbomb1.cbsistatic.com/uploads/scale_small/13/135472/1891761-004charmander.png">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://d2skuhm0vrry40.cloudfront.net/2019/articles/2019-10-31-15-44/pokemon_sword_shield_starters_grookey.jpg/EG11/resize/300x-1/quality/75/format/jpg">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://pokemonletsgo.pokemon.com/assets/img/common/char-eevee.png">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcQIuCM0zB4HkGx85Z8D6EdRVrlGxAUWT1dbwZcZFNzonHle__6Uhg&s">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcQcvGrOH5M64dkU9a-XC-P5rDkPLABag47e88zGybJtFbWPjsUh&s">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://pokemonletsgo.pokemon.com/assets/img/common/char-pikachu.png">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
<div class="col l3">
<div class="card">
<div class="card-image">
<img src="https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRiOrI-gcZubLIku3Ogxl6SLC2_ZmDgNjrPpk095qBxuxKX8lxmvA&s">
</div>
<div class="card-content">
<p>Add content</p>
</div>
</div>
</div>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="index.js"></script>
</body>
</html>
Output
So now we have something which looks like this. Pretty cool right?
Our exercise would be to read the HTML content, change it and add some html content.
First let's see how we read some HTML content through JQuery? Make sure you have created an index.js file in the root directory and linked it to the index.html. Before that, let's add some Pokemon names manually.
<p class="center name">Squirtle</p>
<p class="center name">Charmander</p>
Output
My Pokedex
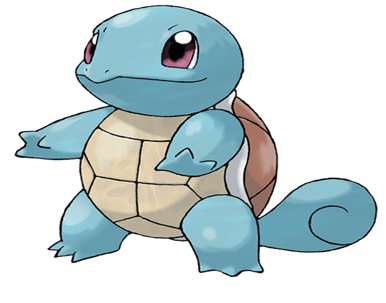
Squirtle
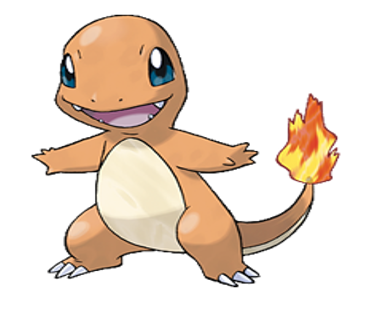
Charmander
Great. Let's see how we can get this content in JQuery,
Index.js:
const names=$('.name');
console.log(names);
We have a reference to all the elements with the class name inside our names variable.
Output
.fn.init(2) [p.center.name, p.center.name, prevObject: k.fn.init(1)]
0: p.center.name
1: p.center.name
length: 2
prevObject: k.fn.init [document]
__proto__: Object(0)
As always we get back a JQuery object and inside this we have on the 0th and 1st indices the names of our first two pokemons that we added. Let's see them,
console.log(names[0],names[1]);
Output
<p class="center name">Squirtle</p>
<p class="center name">Charmander</p>
Now we get these elements back. To get the name of our pokemons, we'll actually have to target the text content inside these elements. In JQuery we do this by,
names.text();
Output
SquirtleCharmander
The text() method returns us the text content of that element. Okay, I just realized I need to change the title of our site a bit, so let's do this now,
const title=$('h1');
console.log(title.text('My Awesome Pokedex'));
Output
My Awesome Pokedex
We can specify what the text content has to be for that targeted element by passing it as a string inside the text() method. It will completely overwrite the current text content with the one we just added. We can also see the HTML inside an element using the html() method,
title.html();
Output
"My Awesome Pokedex"
We can also use this method to add some HTML to that element. Let's use these two methods to set names of some pokemons. Before we do that, let's give a unique id to each pokemon and also write some HTML for some of the cards,
<p class="center name">Squirtle</p>
...
<div class="card-content" id="1">
Now let's add some names using the text() method. I think the 7th pokemon on the pokedex is Pikachu so let's name this little guy. Make sure you have given it a class of center and name.
<p class="center name">Add content</p>
index.js:
$('#7 p').text('Pikachu');
Output
“Pikachu”
The pikachu card has the pikachu name on it
Let's add some html to our jigglypuff now.
index.js:
$('#5').html('<p class="center name">Jigglypuff</p>');
Output
My Pokedex
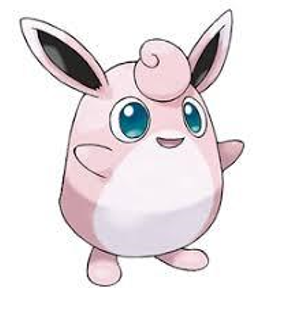
Jigglypuff
Our JigglyPuff has a name now! Great. But wait, was it really Jigglypuff? No, it’s Wigglytuff! We can correct this using the text() method.
index.js:
$('#5 p').text('Wigglytuff');
Now we get the Wigglytuff, just as it should be! However, what if you wanted to update every element on the page and set it’s inner text. Assigning a unique id, getting a reference to all those elements would be tedious. We can avoid this by using the vanilla JS property which is also available in JQuery,
names[3].textContent="Eevee";
Output
My Pokedex
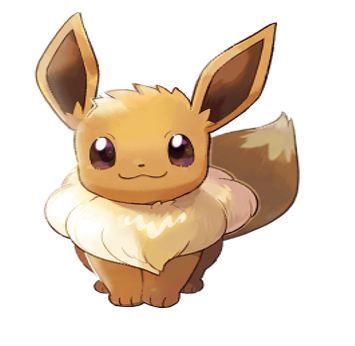
Eevee
This way you can easily update the name or even the HTML for all the cards. As an exercise, try naming all these Pokemon using the first method as well as the second method. Complete this mini pokedex so we can write some more JQuery in the next article and learn something more while we complete this app.
Advertisement
Advertisement