Home »
Android
Android Services Example
In this tutorial, we are going to learn about services in Android, this tutorial contains definition, types of services, methods which will be used in the example and an example of android services.
Submitted by Shamikh Faraz, on April 28, 2018
A service is a component that runs in the background for supporting different types of operations that are long running. The user is not interacted with these. These perform task even if application is destroyed. Examples include handling of network transactions, interaction with content providers, playing music.
This is of two types:
- Started
- Bound
1) Started Service
A service is started when startService() method is called by an activity. It continues to run in the background. It is stopped when stopService() method is called.
2) Bound Service
A service is bound when bindService() method is called by an activity. When unbindService() method is called the component is unbind.
Example of Started and Bound services
For instance I play audio in background, startService() method is called. When I try to get information of current audio file, I shall bind that service that provides information regarding current audio.
Android Services Life Cycle
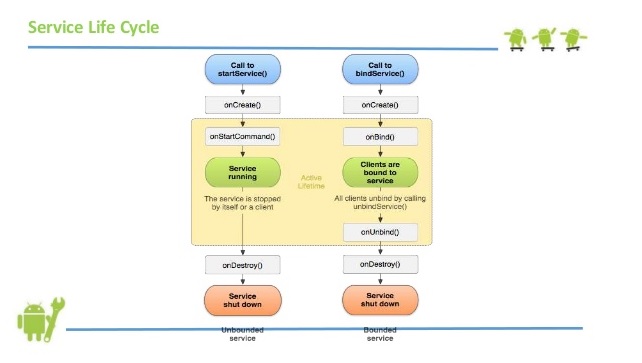
Image source: Google
Methods used in Services
- onStartCommand()
This method is called, when an activity wish to start a service by calling startService().
- onBind()
This method is called when another component such as an activity wish to bind with the service by calling bindService().
- onUnbind()
This method is called, when all components such as clients got disconnected from an interface.
- onRebind()
This method is called, when new clients connect to service.
- OnCreate()
This method is called when the service is first created using onStartCommand() or onBind().
- onDestroy()
This method is called when the service is being destroyed.
Example - Draw three buttons to start, stop and move to next page.
1) XML File: activity_main
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<Button
android:id="@+id/buttonStart"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="19dp"
android:text="Start Your Service" />
<Button
android:id="@+id/buttonStop"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/buttonNext"
android:layout_alignRight="@+id/buttonStart"
android:layout_marginBottom="35dp"
android:text="Stop Your Service" />
<Button
android:id="@+id/buttonNext"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/buttonStop"
android:layout_centerVertical="true"
android:text="Next Activity" />
</android.support.constraint.ConstraintLayout>
Main Activity includes startService() and stopService() methods to start and stop the service.
2) Java File: MainActivity.java
package com.example.faraz.testing;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
public class MainActivity extends Activity implements OnClickListener {
Button buttonStart, buttonStop,buttonNext;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
buttonStart = (Button) findViewById(R.id.buttonStart);
buttonStop = (Button) findViewById(R.id.buttonStop);
buttonNext = (Button) findViewById(R.id.buttonNext);
buttonStart.setOnClickListener(this);
buttonStop.setOnClickListener(this);
buttonNext.setOnClickListener(this);
}
public void onClick(View src) {
switch (src.getId()) {
case R.id.buttonStart:
startService(new Intent(this, MyService.class));
break;
case R.id.buttonStop:
stopService(new Intent(this, MyService.class));
break;
case R.id.buttonNext:
Intent intent=new Intent(this,NextPage.class);
startActivity(intent);
break;
}
}
}
You have to create raw folder in your res directory for "Myservice.java" activity.
MyService.java includes implementation of methods associated with Service based on requirements.
3) MyService.java
package com.example.faraz.testing;
import android.app.Service;
import android.content.Intent;
import android.media.MediaPlayer;
import android.os.IBinder;
import android.widget.Toast;
public class MyService extends Service {
MediaPlayer myPlayer;
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onCreate() {
Toast.makeText(this, "Service Created", Toast.LENGTH_LONG).show();
myPlayer = MediaPlayer.create(this, R.raw.sun);// paste your audio in place of sun file
myPlayer.setLooping(false); // Set looping
}
@Override
public void onStart(Intent intent, int startid) {
Toast.makeText(this, "Service Started", Toast.LENGTH_LONG).show();
myPlayer.start();
}
@Override
public void onDestroy() {
Toast.makeText(this, "Service Stopped", Toast.LENGTH_LONG).show();
myPlayer.stop();
}
}
4) XML File: activity_nextpage.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="96dp"
android:layout_marginTop="112dp"
android:text="Next Page" />
</LinearLayout>
5) NextPage.java
package com.example.faraz.testing;
import android.app.Activity;
import android.os.Bundle;
public class NextPage extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_next);
}
}
Output
After executing your code on your virtual device, you get following output.